Lite Web SDK (Enrollment)
Recommended SDKWe recommend using the Web Seamless SDK for a smooth integration experience. This option provides a flexible payment solution with pre-built UI components and customization options.
Follow this step-by-step guide to implement and enable Yuno's Lite Web SDK enrollment functionality in your application.
Step 1: Include the library in your project
Before proceeding with the Lite SDK implementation, please refer to the Yuno SDK Integration Guide for detailed instructions on how to properly integrate the SDK into your project.
The integration guide provides three flexible methods:
- Direct HTML script inclusion
- Dynamic JavaScript injection
- NPM module installation
Choose the integration method that best suits your development workflow and technical requirements. After completing the SDK integration, you can proceed with the following steps to implement the lite enrollment functionality.
TypeScript LibraryIf you are using TypeScript, Yuno provides a library that you can use to see all available methods available in the Yuno Web SDK.
Step 2: Initialize SDK with the public key
In your JavaScript application, create an instance of the Yuno
class by providing a valid PUBLIC_API_KEY. Check the Get your API credentials guide if you do not have your credentials. In the example below, the initialized class is attributed to the yuno
constant.
const yuno = await Yuno.initialize(PUBLIC_API_KEY)
Step 3: Create a customer session and an enrollment payment method object
Before continuing with the process, you will need to create a customer session and a payment method object to use in the setup of your SDK Lite integration for enrollment. While creating the payment method object, you will need to define which one is going to be available for your customer to enroll (in the case of SDK Lite, only CARD is available).
VerifyIn case you want to verify cards (zero value authorization) before the enrollment, you can complete the
verify
struct while defining the payment method object for the customer session.
Step 4: Mount the enrollment lite
The configuration and mounting are done in the same step for the Enrollment Lite. To do it, use the yuno.mountEnrollmentLite
function and provide the necessary parameters. The following table lists all parameters and their descriptions.
Parameter | Description |
---|---|
| Refers to the current payment's customer session. Example: |
| This parameter specifies the country for which the payment process is being set up. Use an |
| Defines the language to be used in the payment forms. You can set it to one of the available language options: |
| Control the visibility of the Yuno loading/spinner page during the payment process. |
| Required to receive notifications about server calls or loading events during the payment process. |
| Specifies the HTML element where you want to mount the Yuno SDK. The SDK will be rendered within this element. |
| Define specific settings for the credit card form. |
| Define a callback to run after the enrollment process ends. It is called with |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| Enable the issuer's form (bank list). |
| Provide custom text for payment form buttons to match your application's language or branding. |
| Enables you to ensure that all card transactions are processed as credit only. This option is helpful in markets where cards can act as both credit and debit. To enable, set the |
The next code block presents an example of the Enrollment Lite parameter configuration and mounting.
yuno.mountEnrollmentLite({
customerSession: 'e15648b0-fcd5-4799-a14c-cc463ae8a133',
/**
* The complete list of country codes is available on https://docs.y.uno/docs/country-coverage-yuno-sdk
*/
country_code: country,
/**
* Language can be one of en, fr, jp
* Default is browser language
*/
language: 'en',
/**
* Hide or show the Yuno loading/spinner page
* Default is true
* @optional
*/
showLoading: true,
/**
* Required if you'd like to be informed if there is a server call
* @param { isLoading: boolean, type: 'DOCUMENT' | 'ONE_TIME_TOKEN' } data
* @optional
*/
onLoading: (args) => {
console.log(args);
}
/**
* API card
* @optional
*/
card: {
/**
* Mode render card can be step or extends
* Default extends
*/
type: "extends",
/**
* You can edit card form styles
* Only you should write css, then it will be injected into the iframe
* Example
* `@import url('https://fonts.googleapis.com/css2?family=Luckiest+Guy&display=swap');
* .Yuno-front-side-card__name-label {
* color: red !important;
* font-family: 'Luckiest Guy' !important;
* }`
*/
styles: '',
/**
* Show checkbox for save/enroll card
* Default is false
*/
cardSaveEnable: false,
/**
* Custom texts in Card forms buttons
* Example:
*
* texts: {
* cardForm?: {
* enrollmentSubmitButton?: string;
* paymentSubmitButton?: string;
* }
* cardStepper?: {
* numberCardStep?: {
* nextButton?: string;
* },
* cardHolderNameStep?: {
* prevButton?: string;
* nextButton?: string;
* },
* expirationDateStep?: {
* prevButton?: string;
* nextButton?: string;
* },
* cvvStep?: {
* prevButton?: string;
* nextButton?: string;
* }
* }
* }
*/
texts: {},
/**
* Hide or show the document fields into card form
* Default is true
* @optional
*/
documentEnable: true,
},
/**
* Call back is called with the following object
* @param {{
* status: 'CREATED'
* | 'EXPIRED',
* | 'REJECTED',
* | 'READY_TO_ENROLL',
* | 'ENROLL_IN_PROCESS',
* | 'UNENROLL_IN_PROCESS',
* | 'ENROLLED',
* | 'DECLINED',
* | 'CANCELED',
* | 'ERROR',
* | 'UNENROLLED',
* vaultedToken: string,
* }}
*/
yunoEnrollmentStatus: ({ status, vaultedToken}) => {
console.log('status', { status, vaultedToken})
},
/**
* If this is called the SDK should be mounted again
* @param { error: 'CANCELED_BY_USER' | any }
* @optional
*/
yunoError: (error) => {
console.log('There was an error', error)
},
});
continuePayment
return value or null
continuePayment
return value or nullFor payment methods that require merchant-side action (e.g., when the payment provider requires a redirect URL in a webview), the await yuno.continuePayment()
method will return either an object with the following structure or null:
{
action: 'REDIRECT_URL'
type: string
redirect: {
init_url: string
success_url: string
error_url: string
}
} | null
When the method returns an object, it allows you to handle your application's payment flows that require custom redirect handling. When it returns null, no additional merchant-side action is needed.
Demo AppIn addition to the code examples provided, you can access the Demo App for a complete implementation of Yuno SDKs or go directly to the HTML and JavaScript checkout demos available on GitHub.
Complementary features
Yuno Web SDK provides additional services and configurations you can use to improve customers' experience:
Loader
Control the use of the loader.
Parameter | Description |
---|---|
showLoading | You can hide or show the Yuno loading/spinner page. Enabling this option ensures that the loading component remains displayed until either the hideLoader() or continuePayment() function is called. The default value is true. |
yuno.startCheckout({
showLoading: true,
})
Mode of form rendering
Parameter | Description |
---|---|
| This parameter is optional. It determines the mode in which the payment forms will be displayed. |
| |
| |
| Required only if the type is |
| |
|
yuno.startCheckout({
renderMode: {
/**
* Type can be one of `modal` or `element`
* By default the system uses 'modal'
* It is optional
*/
type: 'modal',
/**
* Element where the form will be rendered.
* It is optional
* Can be a string (deprecated) or an object with the following structure:
* {
* apmForm: "#form-element",
* actionForm: "#action-form-element"
* }
*/
elementSelector: {
apmForm: "#form-element",
actionForm: "#action-form-element"
}
},
})
Below, you will find screenshots presenting the difference between the render modes modal
and elements
for the payment method list.
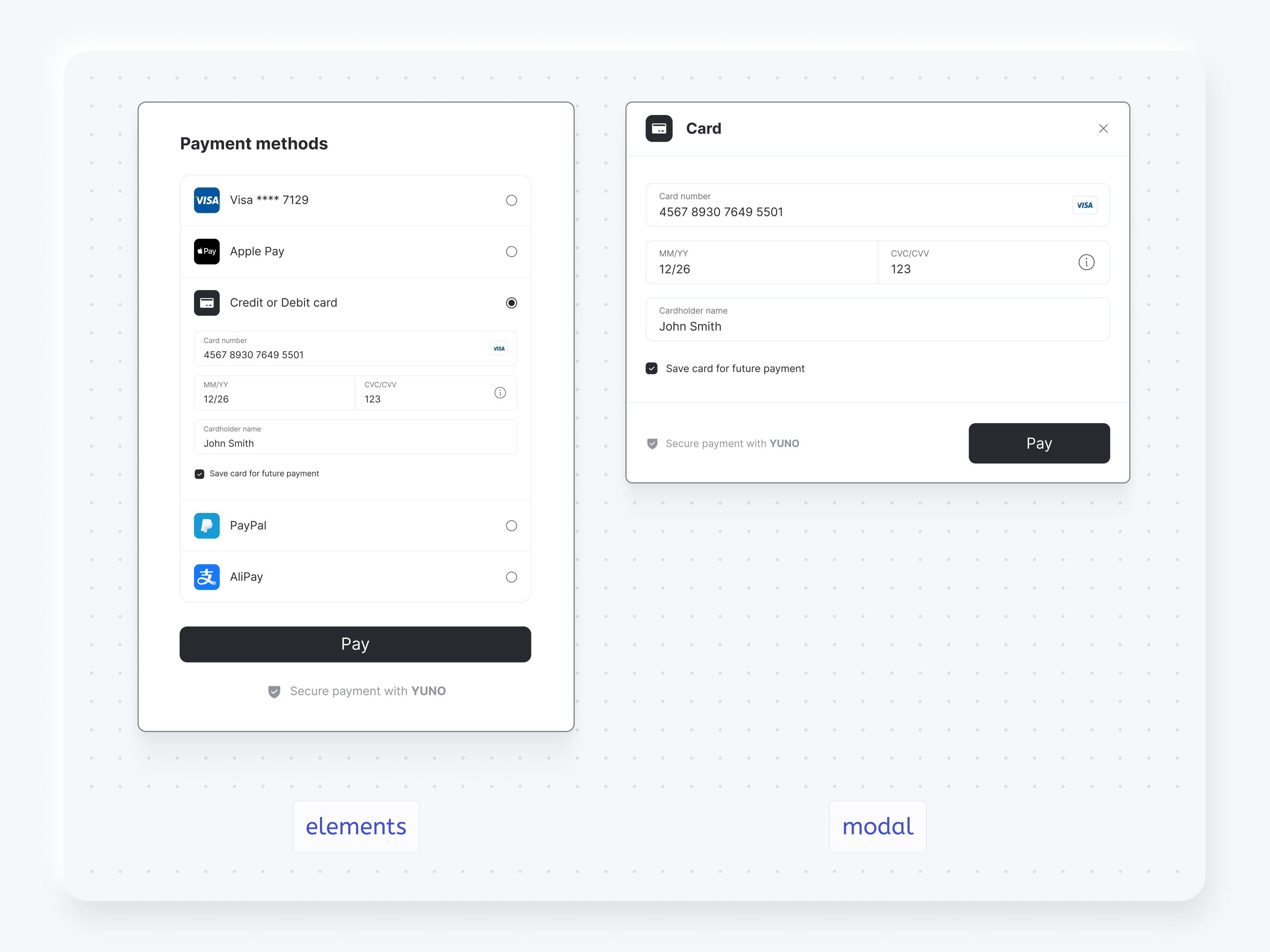
You also can choose one of the render options for the card form, step
and extends
:
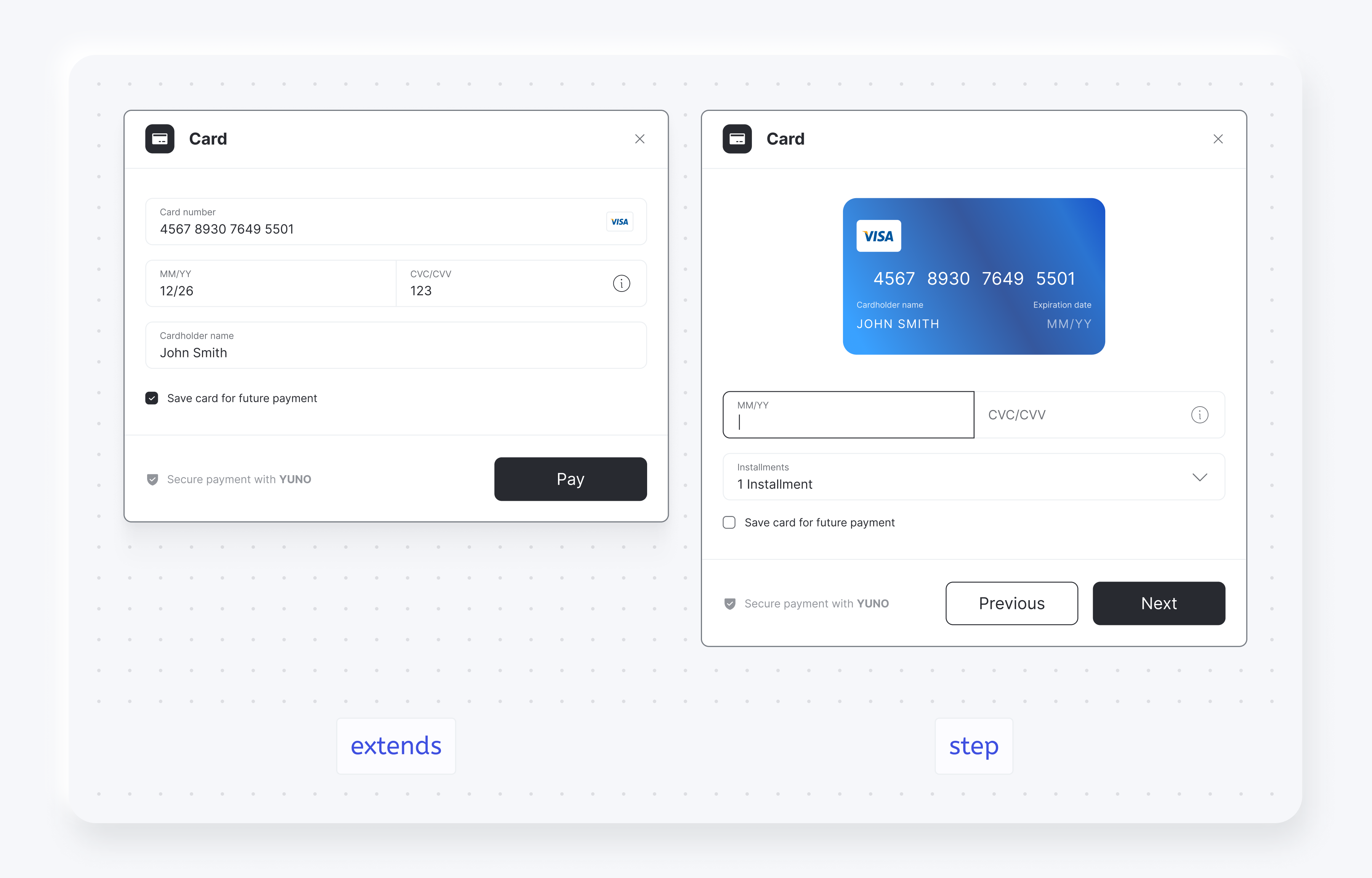
Card form configurations
Parameter | Description |
---|---|
| Define specific settings for the credit card form: |
| |
| |
|
yuno.startCheckout({
card: {
type: "extends",
styles: '',
texts: {}
},
})
Text payment form buttons
Parameter | Description |
---|---|
texts | Provide custom text for payment form buttons to match your application's language or branding. |
yuno.startCheckout({
texts: {
customerForm?: {
submitButton?: string;
}
paymentOtp?: {
sendOtpButton?: string;
}
}
})
SDK customizations
Use the SDK Customizations to change the SDK appearance to match your brand.
What's next?
You can check the additional configurations from the Lite SDK by accessing the Complementary Features or the SDK Customizations, which enable you to change the SDK appearance to match your brand.
Updated about 1 month ago