Headless SDK (Payment)
Yuno's Headless SDK gives you full control over the checkout UX and UI without requiring you to be PCI-compliant.
With the Headless SDK, you can make card payments or enroll card information into your customers' accounts. Access the Headless SDK (Enrollment) for more information. This page covers the payment operation.
Use the following buttons to check the guides available.
Payment workflow
The following image describes the complete payment workflow. Below, each step is described in detail.
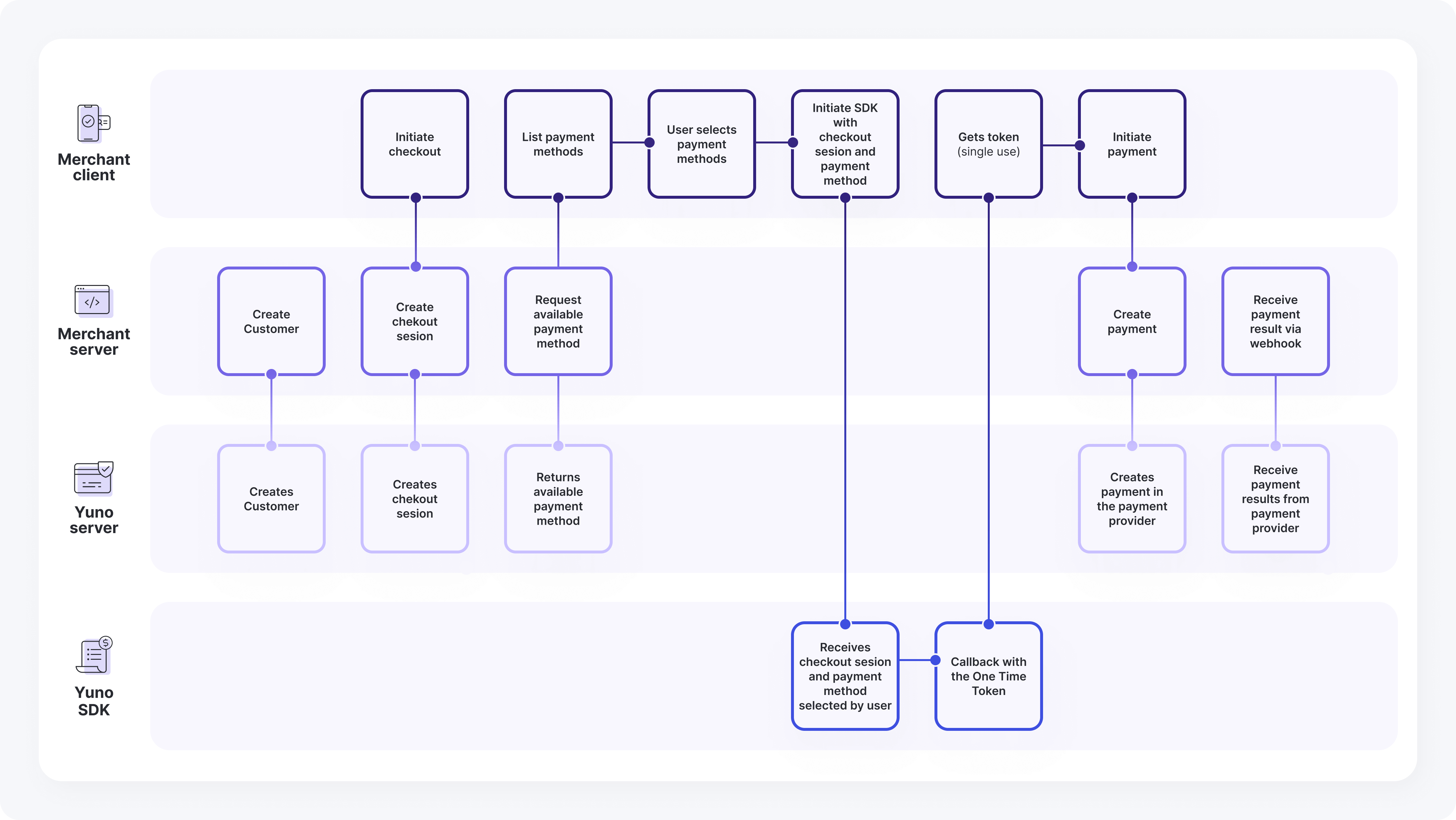
Headless SDK only accepts card payments
The Headless SDK is designed to acept payments using cards. If you need to perform a payment using another payment method, you need to choose use another Yuno integration:
Step 1: Create a customer
First, create a customer. After you create a customer, you can associate payment methods to their account. You can skip this step if you already have a customer ID received from a previous customer creation.
Use the Create Customer endpoint to create new customers and get a customer id
. The customer id
will be used in the following steps.
Step 2: Create a checkout session
Next, create a checkout session. You have to create a new checkout session for every new payment. This session provides access to all available payment methods (previously enrolled or not) for a specific customer.
Use the Create Checkout Session endpoint and provide the customer id
to get a new checkout_session
.
Step 3: Display payment methods
Query the available payment methods using the Retrieve Payment Methods endpoint using the checkout_session
. Show these methods to the customer so they can select their preferred payment method to execute the payment.
If the customer has previously enrolled payment methods, you'll receive them as well. Use the vaulted_token
for these methods to create the One-Time Token and process the payment.
You're responsible for displaying the payment methods and capturing the customer's selection when using the Headless SDK.
Step 4: Implement the SDK and get a One-Time Token
After the customer selects the payment method, you have to initialize the Headless SDK to get a One-Time Token before creating the payment.
To initialize Yuno's Headless SDK, you have to provide your API credentials and the checkout_session
. Follow these steps to complete the process:
- Include the library in your project.
- Initialize the SDK with the public key.
- Start the checkout process by calling
yuno.apiClientPayment()
with your configuration. - Collect the user information and generate the One-Time Token using the
apiClientPayment.generateToken
function.
PCI compliance
You don't need to be PCI compliant when using the Headless SDK. However, you should not store card data, except for the token provided by the SDK, otherwise, you must comply with PCI standards.
For more information on how to initiate Yuno's SDK, refer to one of the following pages according to the corresponding platform:
Step 5: Create the payment
With the One-Time Token, create the payment. This process gathers all order details, including customer specifics, total amount, currency, products, and shipping details. Use the Create Payment endpoint, informing the one_time_token
.
The response from Create Paymentwill include the sdk_action_required
, which defines if additional actions are required based on the payment type:
- Synchronous Payment: If
sdk_action_required
isfalse
, the payment is complete. - Asynchronous Payment: If
sdk_action_required
istrue
, additional actions are required to complete the payment. Use thecontinuePayment
function to complete the payment. See the instructions in Step 6.
Status
During integration, use the payment status
and sub_status
as your primary reference for the payment's state. Since a payment might have multiple associated transactions, concentrating on the payment status
/sub_status
ensures you're informed of the most recent state. This provides a clear basis for decision-making regardless of the number of transactions involved.
Step 6: Continue payment (if needed)
If sdk_action_required
is true, call continuePayment()
to display additional screens or steps for the customer.
Yuno recommends you incorporate the continuePayment()
method because some asynchronous payment methods demand additional action from the customer for completion
Step 7: Receive payment result through webhook
Yuno recommends configuring Webhooks in your dashboard. Webhooks are the best way to ensure your system is up-to-date with payment progress and status. Since the event notifications trigger automatically, your system won't need to perform recurrent requests to Yuno.
Enroll a credit card while paying
With the Headless SDK, you can save credit/debit cards for future purchases with the same payment request without the enrollment integration. You can obtain the vaulted token while executing the apiClientPayment.generateToken
function in Step 4.
Provide a checkbox on your checkout for users to choose if they want to save their card for future use. If the user selects this option, set payment_method.card.save = true
when calling the apiClientPayment.generateToken
function. You'll receive the vaulted_token
in the function response.
To enroll alternative payment methods, see the Lite SDK (Enrollment) page.
After enrolling in a payment method, you can use the vaulted token to perform payments. To access information about the payment methods enrolled by each user, you can use one of the following endpoints:
Using a vaulted token
Even if the user selects an enrolled payment method, Yuno recommends using the SDK to tokenize the information instead of directly using the vaulted token with Yuno's API. This approach provides several benefits:
- Support 3DS: Enhanced security for online payments.
- Fraud Screening: Better protection against fraudulent transactions.
- Collect Required Information: Gather additional fields required by the provider if necessary.
To implement this, send the vaultedToken
when mounting the SDK. The SDK will handle the rest. If the payment method requires an extra step (such as a 3DS challenge), use the yuno.continuePayment()
method. This method handles any required redirections and works for both enrolled and regular payment methods that need additional customer actions.
Updated 6 months ago