Lite SDK (Payment Web)
Follow this step-by-step guide to implement and enable Yuno's Lite Web SDK functionality in your application.
Step 1: Include the library in your project
Before proceeding with the Lite SDK implementation, please refer to the Yuno SDK Integration Guide for detailed instructions on how to properly integrate the SDK into your project.
The integration guide provides three flexible methods:
- Direct HTML script inclusion
- Dynamic JavaScript injection
- NPM module installation
Choose the integration method that best suits your development workflow and technical requirements. After completing the SDK integration, you can proceed with the following steps to implement the lite checkout functionality.
TypeScript library
If you are using TypeScript, Yuno provides a library that you can use to see all available methods available in the Yuno Web SDK.
Step 2: Initialize SDK with the public key
In your JavaScript application, create an instance of the Yuno
class by providing a valid PUBLIC_API_KEY. Check the Get your API credentials guide.
Like the example below, use the initialized class that is attributed to the yuno
constant.
const yuno = await Yuno.initialize(PUBLIC_API_KEY)
Step 3: Start the checkout process
You will start the checkout process. To do it, use the yuno.startCheckout
function and provide the necessary parameters.
The following table lists all required parameters and their descriptions. For optional parameters, go to Complementary Features.
Parameter | Description |
---|---|
checkoutSession | Refers to the current payment's checkout session.Example: '438413b7-4921-41e4-b8f3-28a5a0141638' |
countryCode | This parameter specifies the country for which the payment process is being set up. Use an ENUM value representing the desired country code. You can find the full list of supported countries and their corresponding codes on the Country Coverage page. |
language | Defines the language to be used in the payment forms. You can set it to one of the available language options: es (Spanish), en (English), pt (Portuguese), fil (Filipino), id (Indonesian), ms (Malay), or th (Thai). |
onLoading | Required to receive notifications about server calls or loading events during the payment process. |
showLoading | Control the visibility of the Yuno loading/spinner page during the payment process. By default, it's true . |
issuersFormEnable | Enables the issuer's form. By default, it's true . |
showPaymentStatus | Shows the Yuno Payment Status page. You can use this option when continuing a payment as well. By default, it's true . |
card.isCreditCardProcessingOnly | Enables you to ensure that all card transactions are processed as credit only. This option is helpful in markets where cards can act as both credit and debit. To enable, set the isCreditCardProcessingOnly to true to ensure that all card transactions are processed as credit.This parameter is not required. |
yuno.startCheckout({
checkoutSession: '438413b7-4921-41e4-b8f3-28a5a0141638',
/**
* The complete list of country codes is available on https://docs.y.uno/docs/country-coverage-yuno-sdk
*/
country_code: "FR",
language: 'fr',
showLoading: true,
issuersFormEnable: true,
showPaymentStatus: true,
onLoading: (args) => {
console.log(args);
}
async yunoCreatePayment(oneTimeToken) {
await createPayment({ oneTimeToken, checkoutSession })
yuno.continuePayment({ showPaymentStatus: true })
},
})
Customer and merchant-initiated transactions
Payments can be initiated by the customer (CIT) or by the merchant (MIT). You find more information about their characteristics in Stored credentials.
The step-by-step presented on this page refers to a customer-initiated transaction without the recurrence option. Typically, it's used in one-time online purchases, in-store purchases, ATM withdrawals, etc.
To learn how to use the Lite SDK to perform MIT operations, access the Merchant-initiated transactions page.
Rendering mode
By default, Yuno SDK renders as a modal. However, you can specify the element where the SDK will render. For additional information, access the Render mode under the complementary complementary features page.
Step 4: Mount the SDK
Next, you have to mount the SDK, presenting the checkout based on the payment method selected by your customer. Remember, when using the Lite SDK, you're responsible for displaying the payment methods and capturing the customer's selection. Access Lite SDK (Payment) for additional information.
Use the yuno.mountCheckoutLite()
function by selecting an HTML element and using a valid CSS selector (#
, .
, [data-*]
) to display the checkout for the selected payment method.
yuno.mountCheckoutLite({
/**
* can be one of 'PAYPAL' | 'PIX' | 'APPLE_PAY' | 'GOOGLE_PAY' | CARD
*/
paymentMethodType: PAYMENT_METHOD_TYPE,
/**
* Vaulted token related to payment method type.
* Only if you already have it
* @optional
*/
vaultedToken: VAULTED_TOKEN,
})
After mounting the SDK, the selected payment method flow will start automatically.
Step 5: Initiate the payment process
After the user has selected a payment method, remember to call yuno.startPayment()
to initiate the payment flow. Below, you will find an example where yuno.startPayment()
is called when the user clicks on button-pay
:
const PayButton = document.querySelector('#button-pay')
PayButton.addEventListener('click', () => {
yuno.startPayment()
})
Step 6: Get the OTT (one-time token)
Once the customer fills out the requested data in Yuno's payment forms, the SDK provides the one-time token. The configuration function yunoCreatePayment(oneTimeToken)
is then triggered with the one-time token.
yunoCreatePayment(oneTimeToken)
You can also use tokenWithInformation
to receive any additional info the customer gives at checkout, such as installments or document type/number.
yunoCreatePayment(oneTimeToken, tokenWithInformation)
Important
The merchant is responsible for handling the loader. Yuno offers an option to use our loader; however, the merchant can use their own loader and must make the corresponding configurations.
Step 7: Create the Payment
Once you have completed the steps described before, you will be able to create a payment. The back-to-back payment creation must be carried out using the Create Payment endpoint. The merchant should call their backend to create the payment within Yuno, using the one-time token and the checkout session.
Continue method
Yuno requires you integrate the continuePayment
method of the SDK after the payment is created because certain asynchronous payment methods require additional action from the customer to complete it. The API will inform you of this scenario via the sdk_action_required
field of the response, which will be returned as true. The yuno.continuePayment()
function will display the additional screens to the customers, where they can carry out the necessary actions to complete the payment without needing you to handle every scenario.
continuePayment
return value or null
continuePayment
return value or nullFor payment methods that require merchant-side action (e.g., when the payment provider requires a redirect URL in a webview), the await yuno.continuePayment()
method will return either an object with the following structure or null:
{
action: 'REDIRECT_URL'
type: string
redirect: {
init_url: string
success_url: string
error_url: string
}
} | null
When the method returns an object, it allows you to handle your application's payment flows that require custom redirect handling. When it returns null, no additional merchant-side action is needed.
Demo App
In addition to the code examples provided, you can access the Demo App for a complete implementation of Yuno SDKs or go directly to the HTML and JavaScript checkout demos available on GitHub.
Complementary features
Yuno Web SDK provides additional services and configurations you can use to improve customers' experience:
Loader
Control the use of the loader.
Parameter | Description |
---|---|
showLoading | You can hide or show the Yuno loading/spinner page. Enabling this option ensures that the loading component remains displayed until either the hideLoader() or continuePayment() function is called.The default value is true. |
yuno.startCheckout({
showLoading: true,
})
Form of the issuer
Parameter | Description |
---|---|
issuersFormEnable | Through this parameter, you can configure the SDK to enable the issuer's form (bank list). |
yuno.startCheckout({
issuersFormEnable: true,
})
Mode of form rendering
Parameter | Description |
---|---|
renderMode | This parameter is optional. It determines the mode in which the payment forms will be displayed. - type : can be one of modal or element .- elementSelector : Element where the form will be rendered. Only required if type is element . |
elementSelector | Required only if the type is element . Specifies the HTML elements where you want to mount the Yuno SDK. You can specify the elements using one of the following options:- String (Deprecated): Provide the ID or selector of the element where the SDK should be mounted. - Object: Specify the elements for mounting the APM and action forms. You need to provide the element for the apmForm , which is where the APM is displayed, and the element for the actionForm , where the Continue Payment button appears. This button triggers a modal that shows the steps to complete a payment with a provider. For example, with PIX, it displays a QR code. |
yuno.startCheckout({
renderMode: {
/**
* Type can be one of `modal` or `element`
* By default the system uses 'modal'
* It is optional
*/
type: 'modal',
/**
* Element where the form will be rendered.
* It is optional
* Can be a string (deprecated) or an object with the following structure:
* {
* apmForm: "#form-element",
* actionForm: "#action-form-element"
* }
*/
elementSelector: {
apmForm: "#form-element",
actionForm: "#action-form-element"
}
},
})
Card form configurations
Parameter | Description |
---|---|
card | Define specific settings for the credit card form: - type : step o extends - styles : You can edit card form styles. Only you should write css, then it will be injected into the iframe.- cardSaveEnable : Show checkbox for save/enroll card. The default value is false.- texts : Custom texts in the Card forms buttons. |
yuno.startCheckout({
card: {
type: "extends",
styles: '',
cardSaveEnable: false,
texts: {}
},
})
Save card for future payments
In addition, you can display a checkbox for saving or enrolling cards using the cardSaveEnable: true
. Below are examples of the checkbox for both card form renders.
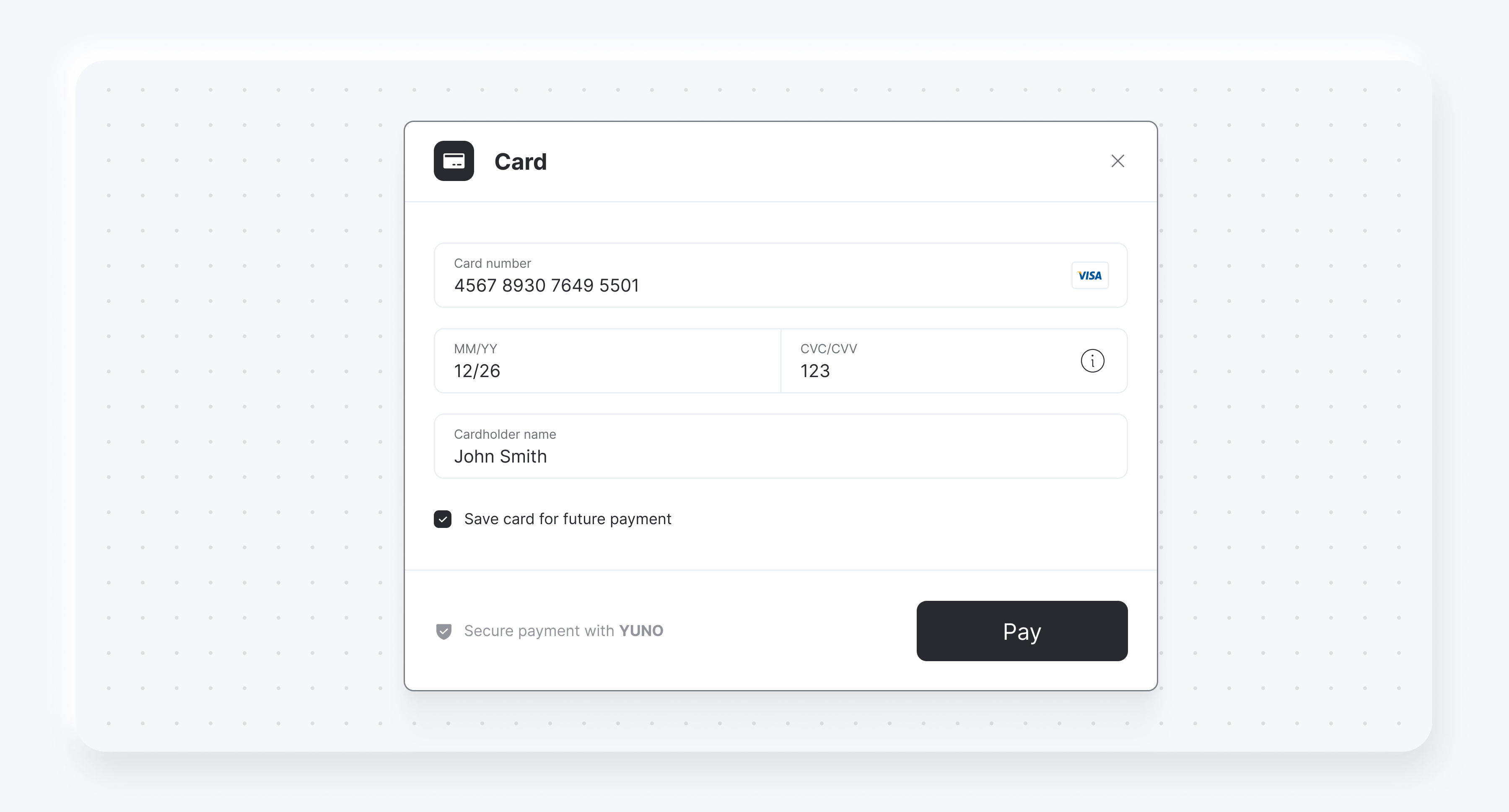
Rendering modes
Below you find screenshots presenting the difference between the following:
- Render modes
modal
andelements
for the payment method list - Render modes
step
andextends
for the credit card form
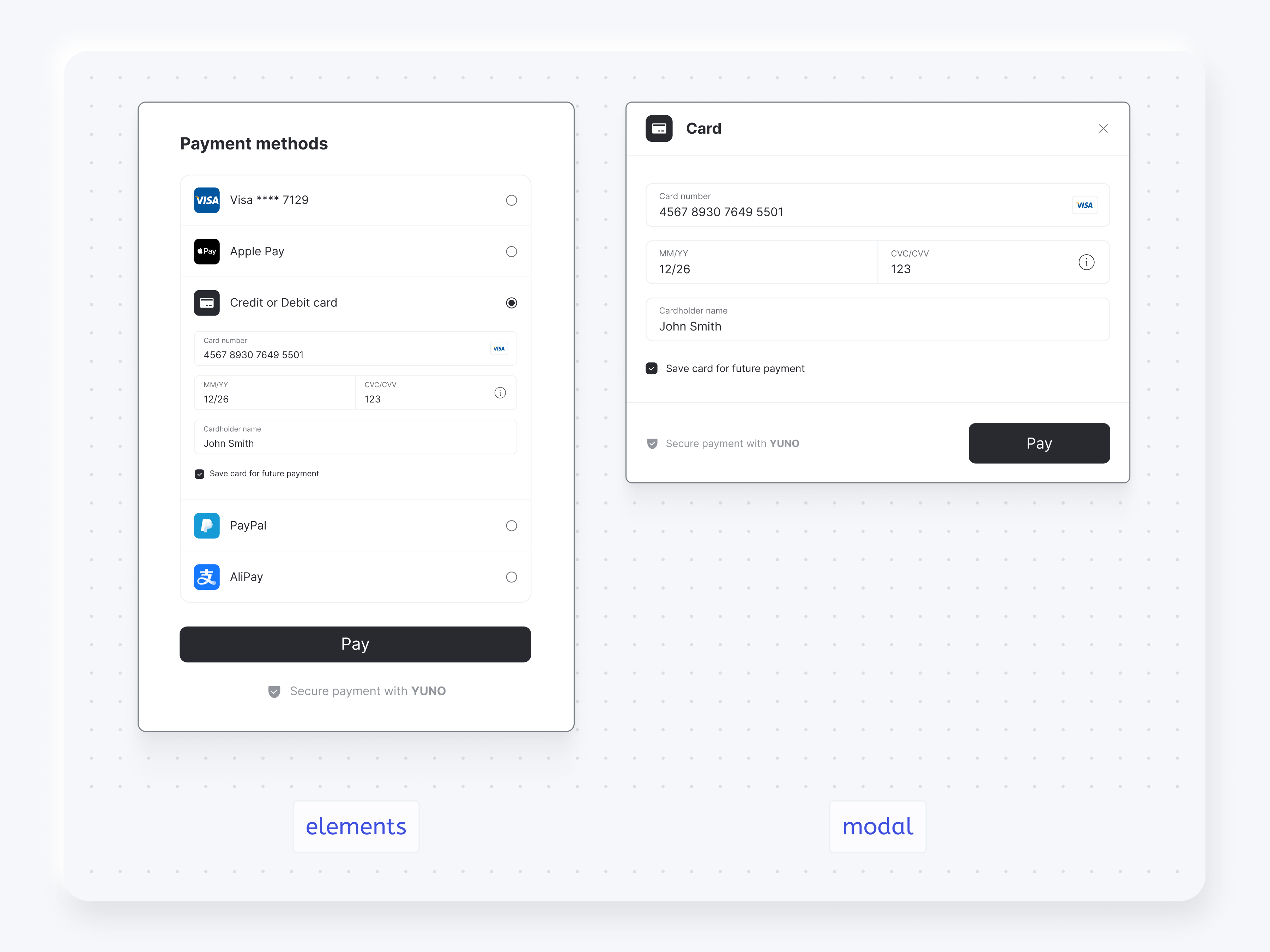
You also can choose one of the render options for the card form, step
and extends
:
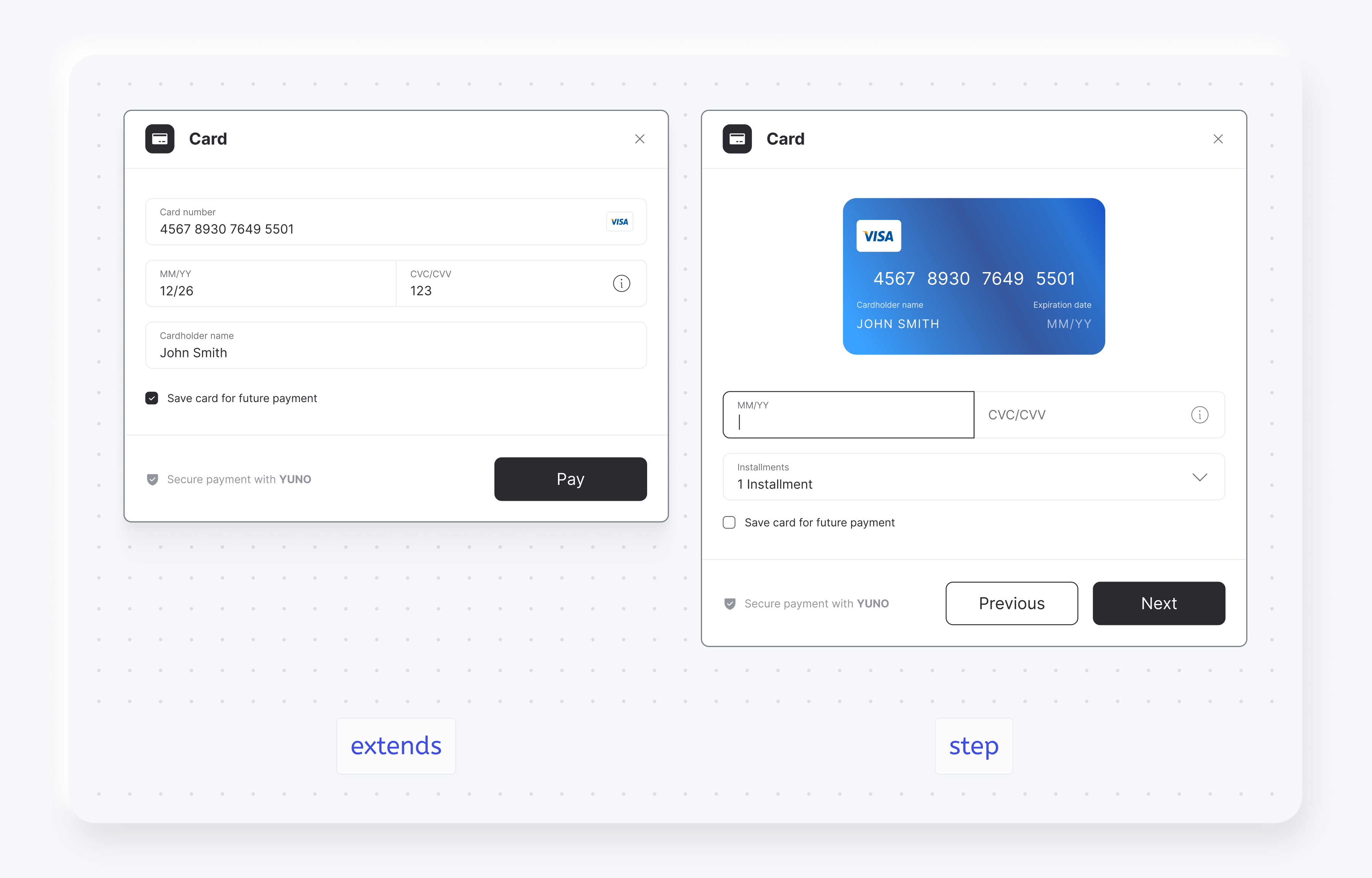
Text payment form buttons
Parameter | Description |
---|---|
texts | Provide custom text for payment form buttons to match your application's language or branding. |
yuno.startCheckout({
texts: {
customerForm?: {
submitButton?: string;
}
paymentOtp?: {
sendOtpButton?: string;
}
}
})
Persist credit card form to retry payments
If a transaction is rejected, you can use the credit card form to retry a payment after the customer has entered the credit card details. To do that, you will need to:
- Add the following parameter while initializing the SDK to persist the credit card form after the one-time use token is created:
yuno.startCheckout({ automaticallyUnmount: false, })
- In case the transaction is rejected, you will need to:
- Execute the method
yuno.notifyError()
to delete the previously entered CVV for the first transaction. - Create a new checkout session and update the SDK with the new one by executing
yuno.updateCheckoutSession(checkoutsession)
- Execute the method
- Continue with the new checkout and one-time use token with the regular payment flow.
Hide Pay button
You can hide the Pay button when presenting the Card or Customer Data Forms. To control this feature, you'll set showPayButton
to false
when starting the checkout with the startCheckout
function. The code block below presents an example of how to hide the payment button:
yuno.startCheckout({
/**
* Hide (false) or show (true) the customer or card form pay button
* @default true
* @optional
*/
showPayButton: false,
})
The following images present examples of the Customer Data Form with and without the Pay button:
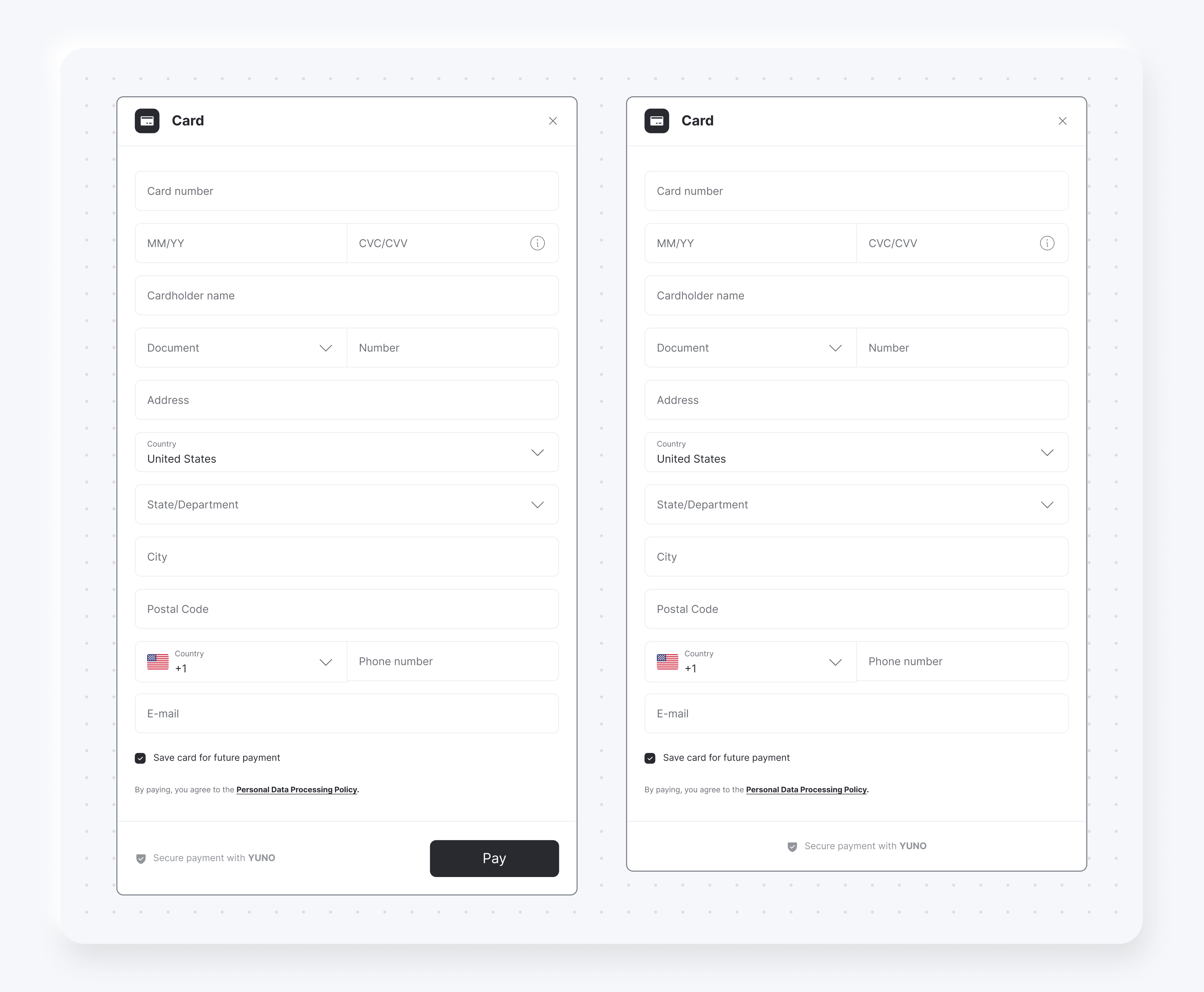
The following images present examples of the Card Form with and without the Pay button:
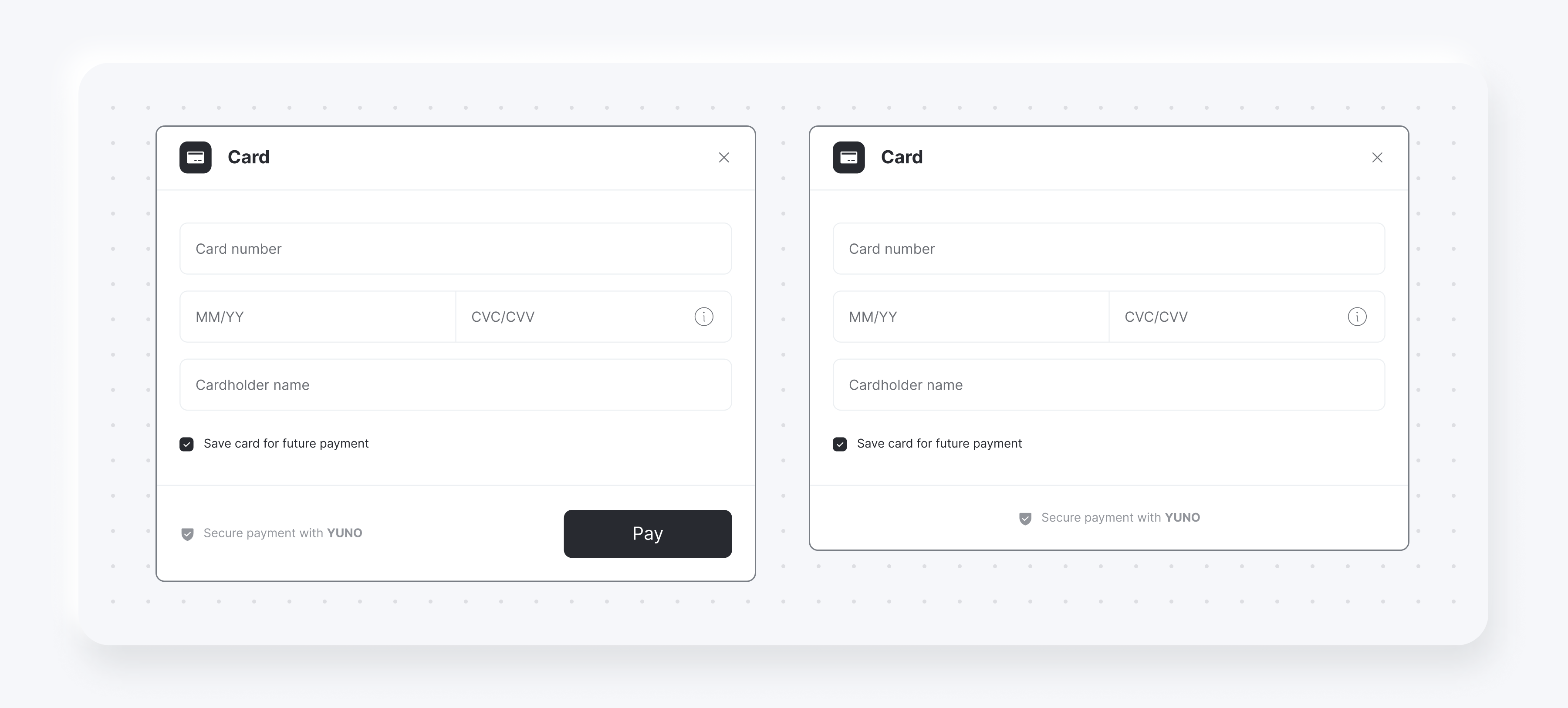
If you hide the Pay button, you will need to start the one-time token creation through your code. To create the one-time token and continue the payment in your backend, call the submitOneTimeTokenForm
function. The code block below presents how to use the submitOneTimeTokenForm
function.
/**
* This function triggers the same functionality that is called when the customer clicks on the pay form button. This approach does not work if you choosed step for rendering mode.
*/
yuno.submitOneTimeTokenForm()
What's next?
Learn how to execute MIT using the Lite SDK, or for additional configurations from the Lite SDK, access Complementary Features. You can also access other functions available on the Yuno Web SDK:
- SDK Customizations: Change the SDK appearance to match your brand
- Payment Status: Update the user about the payment process
- 3DS Setup SDK: Integrate 3DS into your payment flow
Updated 17 days ago