Lite SDK (Enrollment Android)
On this page, you will find all the steps to perform an enrollment using the Lite SDK in your Android project.
Step 1: Include the library in your project
Ensure the Yuno SDK file is included in your project through Gradle. Then, add the repository source using the following code line:
maven { url "https://yunopayments.jfrog.io/artifactory/snapshots-libs-release" }
After, include the code below in the file build.gradle
to add the Yuno SDK dependency to the application.
dependencies {
implementation 'com.yuno.payments:android-sdk:{last_version}'
}
Permissions
Yuno SDK includes, by default, the INTERNET
permission, which is required to make network requests.
<uses-permission android:name="android.permission.INTERNET" />
Step 2: Initialize SDK with the public key
First, you need to retrieve your Public API Keys from the Yuno dashboard.
If you haven't implemented a custom application, create one. In the onCreate()
method of your application class, call the initialize function (Yuno.initialize
) as shown in the example below:
class CustomApplication : Application() {
override fun onCreate() {
super.onCreate()
Yuno.initialize(
this,
"your api key",
config: YunoConfig, // This is a data class to use custom configs in the SDK.
)
}
}
Use the data class YunoConfig
to customize SDK behavior. Include this configuration in the Yuno.initialize
:
data class YunoConfig(
val cardFlow: CardFormType = CardFormType.ONE_STEP, // This is optional, CardFormType.ONE_STEP by default, this is to choose Payment and Enrollment Card flow.
val saveCardEnabled: Boolean = false, // This is to choose if show save card checkbox on cards flows.
val keepLoader: Boolean = false // This is to choose if keep Yuno loading screen until you create and continue with payment, this need an additional step that is shown below.
val language: YunoLanguage? = null, //This is to choose the language of the SDK, if you send null or don't send it, Yuno SDK will take device language.
val isDynamicViewEnabled: Boolean = false, //This is to choose if you want to use dynamic view or not, if you send false or don't send it, Yuno SDK will take false.
)
In the next table, you find the descriptions for each customization option available.
Customization option | Description |
---|---|
cardFlow | It is an optional configuration that defines Payment and Enrollment Card flow. By default, the CardFormType.ONE_STEP option is used. |
saveCardEnabled | Enables the Save card checkbox on card flows. |
keepLoader | Keep Yuno's loading screen until you create and continue with payment. To use this feature, you need to use the function startCompletePaymentFlow() , described in the next sections. |
language | Define the language used to present the SDK. If you don't send or provide a null value, Yuno SDK will use the device language. The available options are: - ENGLISH - SPANISH - PORTUGUESE - INDONESIAN - MALAYSIAN |
isDynamicViewEnabled | Defines if you want to use the dynamic view. In the case you don't provide a value, Yuno SDK will use the FALSE option. |
To ensure that the Yuno loading screen persists until you create and proceed with the payment, you need to use the startCompletePaymentFlow()
function.
You also need to update your manifest to use your application:
<application android:name=".CustomApplication"></application>
Step 3: Enroll a new payment method
To create the enrollment flow, you need to call the initEnrollment
method on the onCreate
method of your activity. This process is required because Yuno's Lite SDK uses it to register the contract to give you the final enrollment state.
fun ComponentActivity.initEnrollment(
callbackEnrollmentState: ((String?) -> Unit)? = null, //Default null | To register this callback is a must to call ```initEnrollment``` method on the onCreate method of activity.
)
To start the enrollment of a new payment method, you need to use the startEnrollment
method. When you call the startEnrollment
method, the enrollment flow of a new payment method will start.
fun Activity.startEnrollment(
customerSession: String,
// The complete list of country codes is available on https://docs.y.uno/docs/country-coverage-yuno-sdk
countryCode: String,
showEnrollmentStatus: Boolean = true, //Optional - Default true
callbackEnrollmentState: ((String?) -> Unit)? = null, // Default null | To register this callback is a must to call ```initEnrollment``` method on the onCreate method of activity.
requestCode: Int, // Optional. Use this option to capture the status when using the onActivityResult
)
Below is a description of the startEnrollment
parameters.
Parameter | Description |
---|---|
customerSession | The session customer associated with the current enrollment process. |
countryCode | Country code where the enrollment is performed. The complete list of supported countries and their country code is available on the Country coverage page. |
showEnrollmentStatus | Indicates whether the enrollment status should be shown. This parameter is optional and defaults to true . |
callbackEnrollmentState | A function that returns the current state of the enrollment process. This parameter is optional and defaults to null . To register this callback, you must call initEnrollment method in the onCreate method of the activity. Check the possible states that can be returned. |
requestCode | It is an optional parameter you must inform if you are going to use the onActivityResult method to capture the enrollment states. |
Callback Enrollment State
The parametercallbackEnrollmentState
is a function that returns the current enrollment process status. Sending this function is not mandatory if you do not need the result.
You can also check the current enrollment state at any moment by using the function AppCompatActivity.enrollmentStatus()
, presented in the following code block. This is an optional action you can take. The functionenrollmentStatus
uses the same parameters as startEnrollment
.
fun AppCompatActivity.enrollmentStatus(
customerSession: String,
countryCode: String,
showEnrollmentStatus: Boolean = false, //Optional - Default false
callbackEnrollmentState: ((String?) -> Unit)? = null, //Optional - You can send again another callback that is gonna override the one you sent on initEnrollment function.
)
Using the function enrollmentStatus
is optional. It isn't a requirement to complete the enrollment process.
If you provide a new callback when calling the function enrollmentStatus
, it will override the callback you set when calling the functioninitEnrollment
.
The possible states are presented in the following code block:
const val ENROLLMENT_STATE_SUCCEEDED = "SUCCEEDED"
const val ENROLLMENT_STATE_FAIL = "FAIL"
const val ENROLLMENT_STATE_PROCESSING = "PROCESSING"
const val ENROLLMENT_STATE_REJECT = "REJECT"
const val ENROLLMENT_STATE_INTERNAL_ERROR = "INTERNAL_ERROR"
const val ENROLLMENT_STATE_CANCELED_BY_USER = "CANCELED"
Using the OnActivityResult
method
OnActivityResult
methodThe onActivityResult method is automatically invoked when an activity returns a result. You can use this option to execute actions whenever the enrollment status changes. To process the enrollment result, follow these steps:
Depricated
The onActivityResult
method is a deprecated solution. If you are performing a new Android integration, Yuno recommends using initEnrollment()
contract, which follows Google's best practices.
If you are using the onActivityResult
method but did not inform a requestCode
when calling the startEnrollment
in Step 3, you must use the YUNO_ENROLLMENT_REQUEST_CODE
provided by Yuno.
- First, override the
onActivityResult
method. It ensures that the hierarchy calls are respected.
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
}
- After, check if the
requestCode
to verify if it corresponds toYUNO_ENROLLMENT_REQUEST_CODE
. Since you are runningYuno
in your app, you can import theYUNO_ENROLLMENT_REQUEST_CODE
to use it.
if (requestCode == YUNO_ENROLLMENT_REQUEST_CODE) {
// Specific enrollment processing
}
- If the
requestCode
matches, you can handle the enrollment result. In this case, you can extract the enrollment state using theYUNO_ENROLLMENT_RESULT
key provided by the Yuno library. You can create aonEnrollmentStateChange
function to handle the state changes.
onEnrollmentStateChange(data?.getStringExtra(YUNO_ENROLLMENT_RESULT))
The following code block presents an example of code to use the OnActivityResult
method to execute functions when the enrollment status changes.
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == YUNO_ENROLLMENT_REQUEST_CODE) {
// Handle the enrollment result
val enrollmentState = data?.getStringExtra(YUNO_ENROLLMENT_RESULT)
onEnrollmentStateChange(enrollmentState)
}
}
fun onEnrollmentStateChange(enrollmentState: String?) {
// Implement your logic to handle different enrollment states here
when (enrollmentState) {
"SUCCEEDED" -> {
// Handle successful enrollment
}
"FAIL" -> {
// Handle failed enrollment
}
// Add other states as needed
}
}
Step 4: Callback enrollment state
To register a callback to get the final enrollment state, it is necessary to call the initEnrollment
method on the onCreate
method of activity.
Complementary features
Yuno Android SDK provides additional services, and you can also check the current enrollment configurations you can use to improve customers' experience. Use the SDK Customizations to change the SDK appearance to match your brand or to configure the loader.
Yuno Android SDK provides additional services and configurations you can use to improve customers' experience:
- Loader: Control the use of the loader.
- You also can choose one of the render options for the card form. Below, you find screenshots presenting the difference between the
cardFormType
ONE_STEP
andSTEP_BY_STEP
.
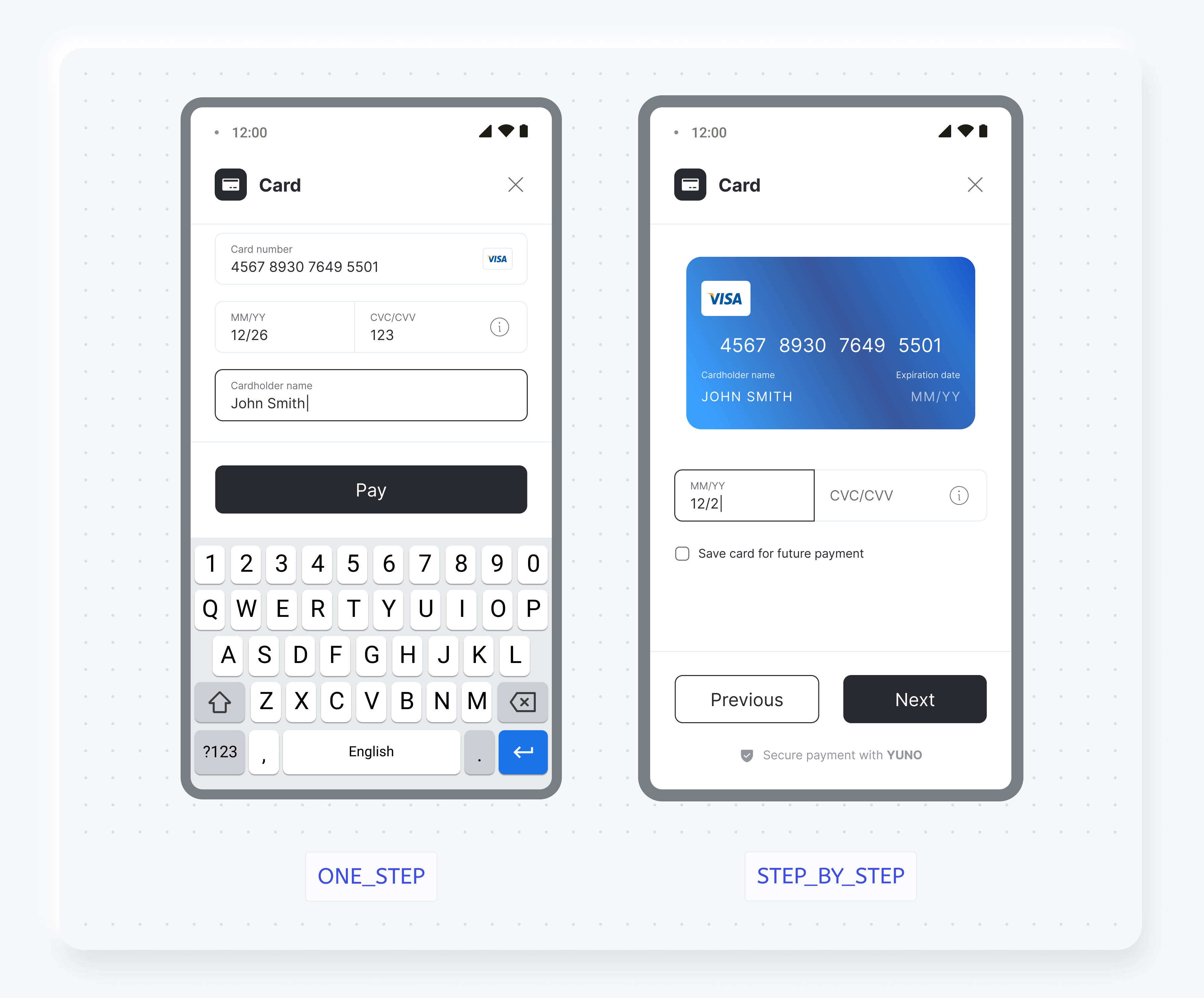
- SDK Customizations: Change the SDK appearance to match your brand.
Demo App
In addition to the code examples provided, you can access the Yuno repository for a complete implementation of Yuno Android SDKs.
Updated 3 months ago